
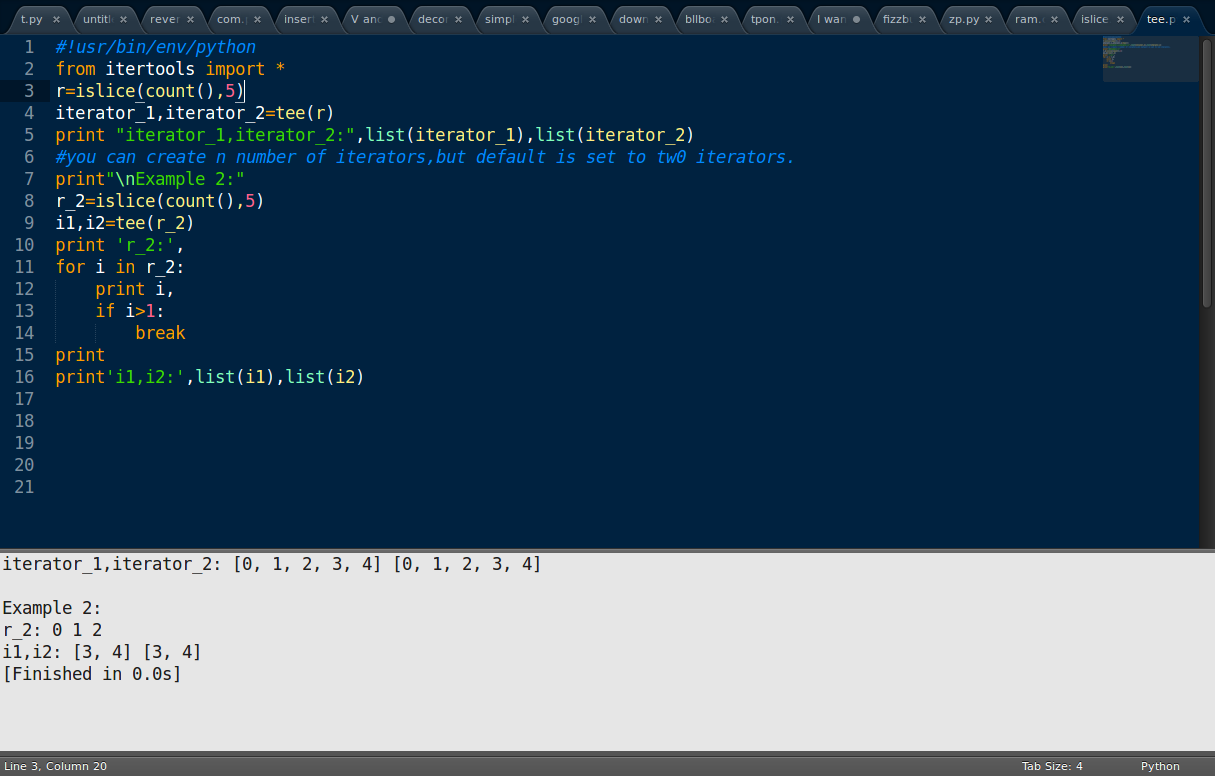
Youll still have to import the itertools module to use it. To return an iterator, the izip() and imap() functions of itertools must be used. The izip() function works the same way, but it returns an iterable object for an increase in performance by reducing memory usage. The module standardizes a core set of fast, memory efficient tools that are useful by themselves or in combination. Question: Write a python program to show the difference in zip() and izip() function Explanation: zip() is a standard library which combines elements into tuples. Each has been recast in a form suitable for Python. This module implements a number of iterator building blocks inspired by constructs from APL, Haskell, and SML. islice () wasnt ported into the built-in namespace of Python 3. Functions creating iterators for efficient looping. They all return iterators and dont require imports.
#From itertools import izip python 3 zip#
It works like the built-in function zip(), except that it returns an. Note: As of Python 3, filter (), map () and zip () are functionally equivalent to Python 2s itertools functions ifilter (), imap () and izip (). So if you want to write 2 variable as a CSV row you can put them in a tuple or list: writer.writerows((variable1,2))Īlso from itertools you can import zip_longest as a more flexible function which you can use it on iterators with different size. izip() returns an iterator that combines the elements of several iterators into tuples. In this case since zip's arguments must support iteration you can not use 2 as its argument. It will return each from the first iterable and then proceed to the next iterable till there are no more iterables left and no more elements to return. Print(timeit('list(zip(range(100), range(100)))', number=500000)) Itertools.chain is used for creating an iterator from two or more iterables: itertools.chain(iterables). Here is a benchmark between zip in Python 2 and 3 and izip in Python 2: except ImportError: Python 2from itertools import iziplongest as ziplongest.

The zip implementation is almost completely copy-pasted from the old izip, just with a few names changed and pickle support added. Which went smoothly, but I when trying to determine the composition I ran into an import problem: python scripts/genkmer.py Traceback (most recent call last): File 'scripts/genkmer. In Python 3 the built-in zip does the same job as itertools.izip in 2.X(returns an iterator instead of a list). Not only because Python’s syntax is elegant, but also due to it has many well-designed built-in modules that help us implement common functionalities efficiently.
